kelly mort
Well-known Member
- Joined
- Apr 10, 2017
- Messages
- 2,169
- Office Version
- 2016
- Platform
- Windows
Hello everyone.
I found this code on stack overflow:
stackoverflow.com
I want to be able to detect the specific app in which the pdf file is opened.
On this line:
How do I go about that?
Thanks in advance.
I found this code on stack overflow:
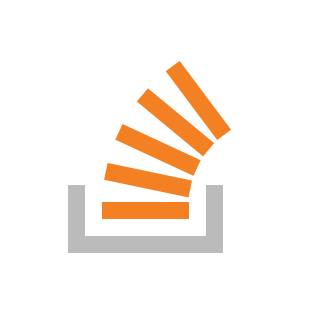
Check if a certain pdf file is open and close it
I use this code to export a pdf file from a word document. Before exporting I need to check first if a file with the same name is already open, and if so close it then export. I tried many things...
I want to be able to detect the specific app in which the pdf file is opened.
On this line:
Code:
Hwnd = FindWindow(vbNullString, "Current Letter Preview.pdf - Adobe Reader")
Code:
Option Explicit
Private Declare Function PostMessage Lib "user32" Alias "PostMessageA" _
(ByVal Hwnd As Long, ByVal wMsg As Long, ByVal wParam As Long, _
lParam As Any) As Long
Private Declare Function FindWindow Lib "user32" Alias "FindWindowA" _
(ByVal lpClassname As String, ByVal lpWindowName As String) As Long
Private Const WM_CLOSE = &H10
Sub Sample()
Dim Hwnd As Long
'~~> Find the window of the pdf file
Hwnd = FindWindow(vbNullString, "Current Letter Preview.pdf - Adobe Reader")
If Hwnd Then
'~~> Close the file
PostMessage Hwnd, WM_CLOSE, 0, ByVal 0&
Else
MsgBox "Pdf File not found"
End If
End Sub
How do I go about that?
Thanks in advance.