looking to grab the last filename in the folder to use the batch number so I can save the next file as batch number +1 in vba.
I used this to pull the files from the folder and it works.
trumpexcel.com
but I really only need the last file so "file 12.xlsm"
is there a way I can grab only the last one or have it decending so I only have to list 1 file?
have a folder with files below
File 1.xlsm
File 2.xlsm
File 3.xlsm
File 4.xlsm
ect
File 10.xlsm
File 11.xlsm
File 12.xlsm
etc
will go up 3 digits though maybe even 4 digits
File 100.xlsm
File 101.xlsm
File 102.xlsm
I used this to pull the files from the folder and it works.
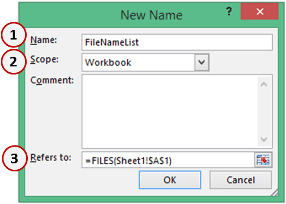
Get the List of File Names from a Folder in Excel (with and without VBA)
This Excel trick uses an old Excel Function FILES() to get the list of all the File Names from a Folder in Excel (or a specific set of file names in Excel)

but I really only need the last file so "file 12.xlsm"
is there a way I can grab only the last one or have it decending so I only have to list 1 file?
have a folder with files below
File 1.xlsm
File 2.xlsm
File 3.xlsm
File 4.xlsm
ect
File 10.xlsm
File 11.xlsm
File 12.xlsm
etc
will go up 3 digits though maybe even 4 digits
File 100.xlsm
File 101.xlsm
File 102.xlsm