Jimmypop
Well-known Member
- Joined
- Sep 12, 2013
- Messages
- 753
- Office Version
- 365
- Platform
- Windows
Hi there
I have the following code to load a image into a userform image control.
The code for GetImageFile(TextBox5.Value) is as follows:
It then looks like this with the image rotated (I tried to rotate the image after loading but none of the code I tried worked... This was covered in a previous post Previous attempts)...
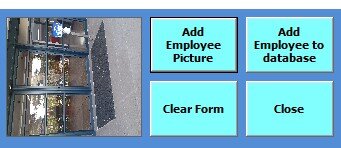
So then I realized that it is to do with the image size which is 1800 x 4000... So then I tried to update my code to the one below to resize the image...(When I do images of this size they load correctly onto the image control and to the sheet)
However, image in Image control is not resizing and now displays as:
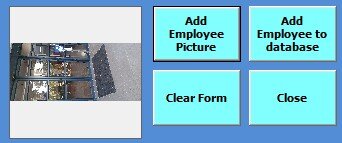
Am I on the correct path to do:
Resize image of 1800 x 4000 to 100 x 100 before it is placed inside the Image Control on the userform...
I have the following code to load a image into a userform image control.
VBA Code:
Private Sub AddEmpPic_Click()
'to place picture inside imageframe
Image1.PictureSizeMode = fmPictureSizeModeStretch
Call GetImageFile(TextBox5.value)
On Error Resume Next
Image1.Picture = LoadPicture(pathToFile)
End Sub
The code for GetImageFile(TextBox5.Value) is as follows:
VBA Code:
Private Function GetImageFile(ByVal Empl As String) As String
With Application.fileDialog(msoFileDialogFilePicker)
.AllowMultiSelect = False
.Filters.Clear
.Filters.Add "JPG", "*.JPG"
.Filters.Add "JPEG File Interchange Format", "*.JPEG"
.Filters.Add "Graphics Interchange Format", "*.GIF"
.Filters.Add "Portable Network Graphics", "*.PNG"
.Filters.Add "Tag Image File Format", "*.TIFF"
.Filters.Add "All Pictures", "*.*"
End With
pathToFile = Application.GetOpenFilename(Title:="Add Employee Image", FileFilter:=UCase(Empl) & " (*.jpg; *.png; *.gif),*.bas;*.png;*.gif")
If pathToFile > "" Then GetImageFile = pathToFile
End Function
It then looks like this with the image rotated (I tried to rotate the image after loading but none of the code I tried worked... This was covered in a previous post Previous attempts)...
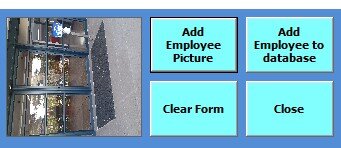
So then I realized that it is to do with the image size which is 1800 x 4000... So then I tried to update my code to the one below to resize the image...(When I do images of this size they load correctly onto the image control and to the sheet)
VBA Code:
Private Sub AddEmpPic_Click()
'to place picture inside imageframe
Me.Image1.PictureSizeMode = fmPictureSizeModeZoom
Me.Image1.Height = 100
Me.Image1.Width = 100
Call GetImageFile(TextBox5.value)
On Error Resume Next
Image1.Picture = LoadPicture(pathToFile)
End Sub
However, image in Image control is not resizing and now displays as:
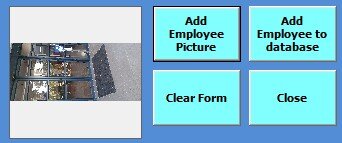
Am I on the correct path to do:
Resize image of 1800 x 4000 to 100 x 100 before it is placed inside the Image Control on the userform...