I'm working with a dynamic table that populates names based on the given weeks data. I have a macro that refreshes and sorts the data as needed but am currently working with the .Resize Range() function to adjust the table based on how the rows populate.
Note: "Login" cells populate based on an IF reference to another sheet:
See table ("Table12") itself below (webdings for privacy)
Prior to including the loop,
(to remove one row)
and
(to add one row)
did exactly what I needed them to.
The trouble started when I wanted the macro to decide which operation to run based on the the last cell in my "Login" column.
The currently included Do Until loop freezes my application but I left it for visibility. The real goal though is to have the macro decide if it should add a row or remove one.
The Code:
Note: "Login" cells populate based on an IF reference to another sheet:
Excel Formula:
=IF(WSSW!AH126=0,"-",WSSW!AH126)
See table ("Table12") itself below (webdings for privacy)
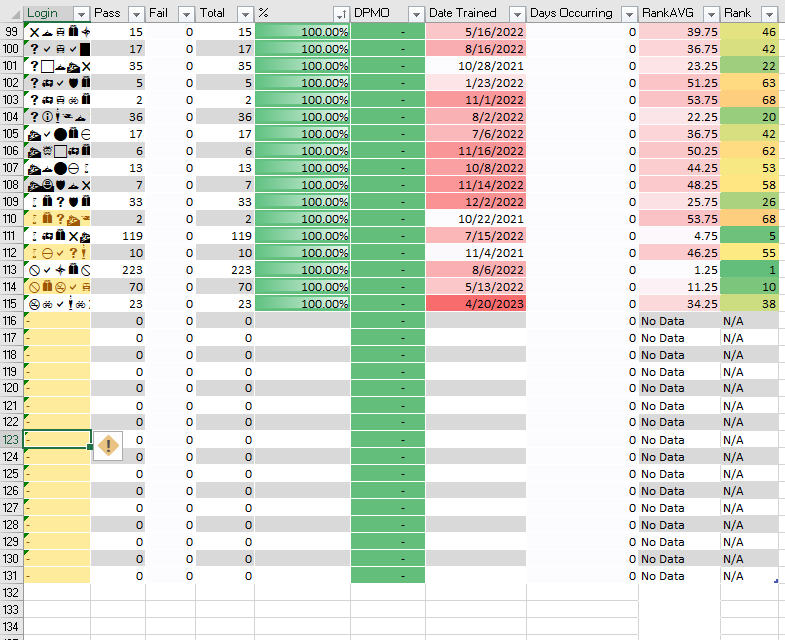
Prior to including the loop,
VBA Code:
mnTbl.Resize Range("$A$3:" & lcol & lrow - 1)
and
VBA Code:
mnTbl.Resize Range("$A$3:" & lcol & lrow + 1)
did exactly what I needed them to.
The trouble started when I wanted the macro to decide which operation to run based on the the last cell in my "Login" column.
The currently included Do Until loop freezes my application but I left it for visibility. The real goal though is to have the macro decide if it should add a row or remove one.
The Code:
VBA Code:
Sub TableDrag()
'
' TableDrag Macro
'
'
Dim MAIN As Worksheet
Set MAIN = ThisWorkbook.Sheets("MAIN")
Dim mnTbl As ListObject
Set mnTbl = MAIN.ListObjects("Table12")
Dim lrow As String
Dim val As String
' Find Last Row
fcol = "A"
lrow = mnTbl.Range.Rows(mnTbl.Range.Rows.Count).Row
val = Range("A" & lrow).Value
'This next part crashes excel. The goal being: IF val equals "-" THEN remove last row AND IF val not equal "-" THEN add row to end of range
Do Until val <> "-"
mnTbl.Resize Range("$A$3:" & lcol & lrow - 1)
Loop
End Sub