Hi everyone
I am trying to make a sheet that has 6 different "markers" (.png images) across the top row (see pic). Each marker is in it's own cell, and are the same size of the cell they cover (102px X 102px). There are 10 copies of each marker stacked on top of each other so that the user can drag multiple markers of the same kind to the user's desired location on a diagram below the top row.
What I am trying to do is this: When the user clicks a reset button, all markers go back into their original cell location, and if any markers were resized by the user they should revert to their original size. (or, make the markers so they cannot be resized. Current code and picture example below. Thank you for your help!
I am trying to make a sheet that has 6 different "markers" (.png images) across the top row (see pic). Each marker is in it's own cell, and are the same size of the cell they cover (102px X 102px). There are 10 copies of each marker stacked on top of each other so that the user can drag multiple markers of the same kind to the user's desired location on a diagram below the top row.
What I am trying to do is this: When the user clicks a reset button, all markers go back into their original cell location, and if any markers were resized by the user they should revert to their original size. (or, make the markers so they cannot be resized. Current code and picture example below. Thank you for your help!
Code:
Private Sub DrawResetButton_Click()
' Move picture to a given cell
With Sheet3
With .Range("B2")
T = .Top
L = .Left
End With
With .Shapes.Item("dome", "dome2") 'this is not working. I cannot figure out how
.Top = T 'to make this apply to two different images, let alone another
.Left = L 'image set (to a different location.
End With
End With
End Sub
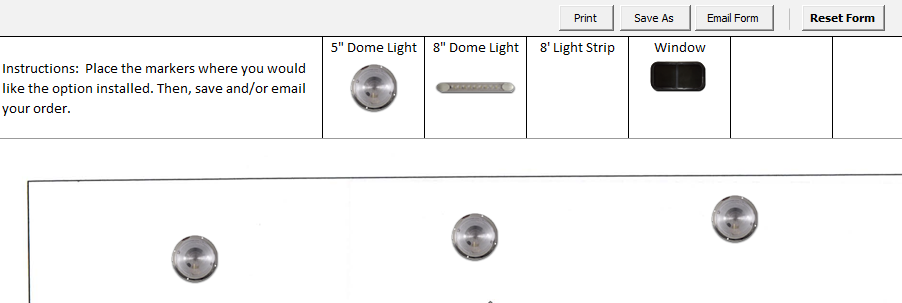