Hello there. As it is probably obvious, I am super green on attempting VBA. But since I have some time, I have been exploring it to streamline processes at work. Here is a small background about my dilemma:
My userform is going to be used to grade employee training with the results being added to an excel spreadsheet. The checkboxes in my form are representative of the different kinds of mistakes they can make and each mistake ideally has a quantity box that will specify how many times each mistake was made if its box is checked. I need both the checkbox caption and the textbox value in one cell, as each row is 1 test for each employee.
Here is a portion of my form as an example:
So far I was able to use a loop with a string to get the checkboxes to put their captions into the single cell using this code:
<code style="margin: 0px; padding: 0px; border: 0px; font-style: inherit; font-variant: inherit; font-weight: inherit; font-stretch: inherit; line-height: inherit; font-family: Consolas, Menlo, Monaco, "Lucida Console", "Liberation Mono", "DejaVu Sans Mono", "Bitstream Vera Sans Mono", "Courier New", monospace, sans-serif; vertical-align: baseline; white-space: inherit;">Dim CheckBox As Control
Dim Mistakes As String, delimiter As String
For Each CheckBox In Me.Frame_Mistakes.Controls
If TypeOf CheckBox Is MSForms.CheckBox Then
If (CheckBox.Value) Then
Mistakes = Mistakes & delimiter & CheckBox.Caption
delimiter = " | "
End If
End If
Next
With Sheet1
.Cells(emptyRow, 4).Value = Mistakes
End With</code>
And I understand that in order to get my output to look like this:
That I need to edit my Mistakes expression to say something like:
<code style="margin: 0px; padding: 0px; border: 0px; font-style: inherit; font-variant: inherit; font-weight: inherit; font-stretch: inherit; line-height: inherit; font-family: Consolas, Menlo, Monaco, "Lucida Console", "Liberation Mono", "DejaVu Sans Mono", "Bitstream Vera Sans Mono", "Courier New", monospace, sans-serif; vertical-align: baseline; white-space: inherit;">Mistakes = Mistakes & delimiter & Checkbox.Caption & "(x" & TextBox.text & ")"</code>
However, I'm not entirely sure how to get my checkboxes to acknowledge their respective textboxes so that the output captions are with the textbox that is assigned to it. I would also like to limit the character size of the textbox to be 2 and force it to only allow numbers (I assume this will be some kind of isnumeric expression).
I have ensured that the checkbox number matches the textbox number for each mistake on my userform (if that helps).
If anyone could offer some help, I would be forever grateful!
My userform is going to be used to grade employee training with the results being added to an excel spreadsheet. The checkboxes in my form are representative of the different kinds of mistakes they can make and each mistake ideally has a quantity box that will specify how many times each mistake was made if its box is checked. I need both the checkbox caption and the textbox value in one cell, as each row is 1 test for each employee.
Here is a portion of my form as an example:
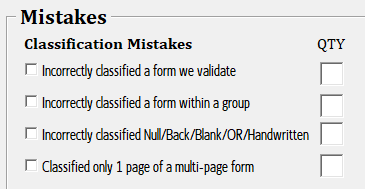
So far I was able to use a loop with a string to get the checkboxes to put their captions into the single cell using this code:
<code style="margin: 0px; padding: 0px; border: 0px; font-style: inherit; font-variant: inherit; font-weight: inherit; font-stretch: inherit; line-height: inherit; font-family: Consolas, Menlo, Monaco, "Lucida Console", "Liberation Mono", "DejaVu Sans Mono", "Bitstream Vera Sans Mono", "Courier New", monospace, sans-serif; vertical-align: baseline; white-space: inherit;">Dim CheckBox As Control
Dim Mistakes As String, delimiter As String
For Each CheckBox In Me.Frame_Mistakes.Controls
If TypeOf CheckBox Is MSForms.CheckBox Then
If (CheckBox.Value) Then
Mistakes = Mistakes & delimiter & CheckBox.Caption
delimiter = " | "
End If
End If
Next
With Sheet1
.Cells(emptyRow, 4).Value = Mistakes
End With</code>
And I understand that in order to get my output to look like this:

That I need to edit my Mistakes expression to say something like:
<code style="margin: 0px; padding: 0px; border: 0px; font-style: inherit; font-variant: inherit; font-weight: inherit; font-stretch: inherit; line-height: inherit; font-family: Consolas, Menlo, Monaco, "Lucida Console", "Liberation Mono", "DejaVu Sans Mono", "Bitstream Vera Sans Mono", "Courier New", monospace, sans-serif; vertical-align: baseline; white-space: inherit;">Mistakes = Mistakes & delimiter & Checkbox.Caption & "(x" & TextBox.text & ")"</code>
However, I'm not entirely sure how to get my checkboxes to acknowledge their respective textboxes so that the output captions are with the textbox that is assigned to it. I would also like to limit the character size of the textbox to be 2 and force it to only allow numbers (I assume this will be some kind of isnumeric expression).
I have ensured that the checkbox number matches the textbox number for each mistake on my userform (if that helps).
If anyone could offer some help, I would be forever grateful!